State Management in React
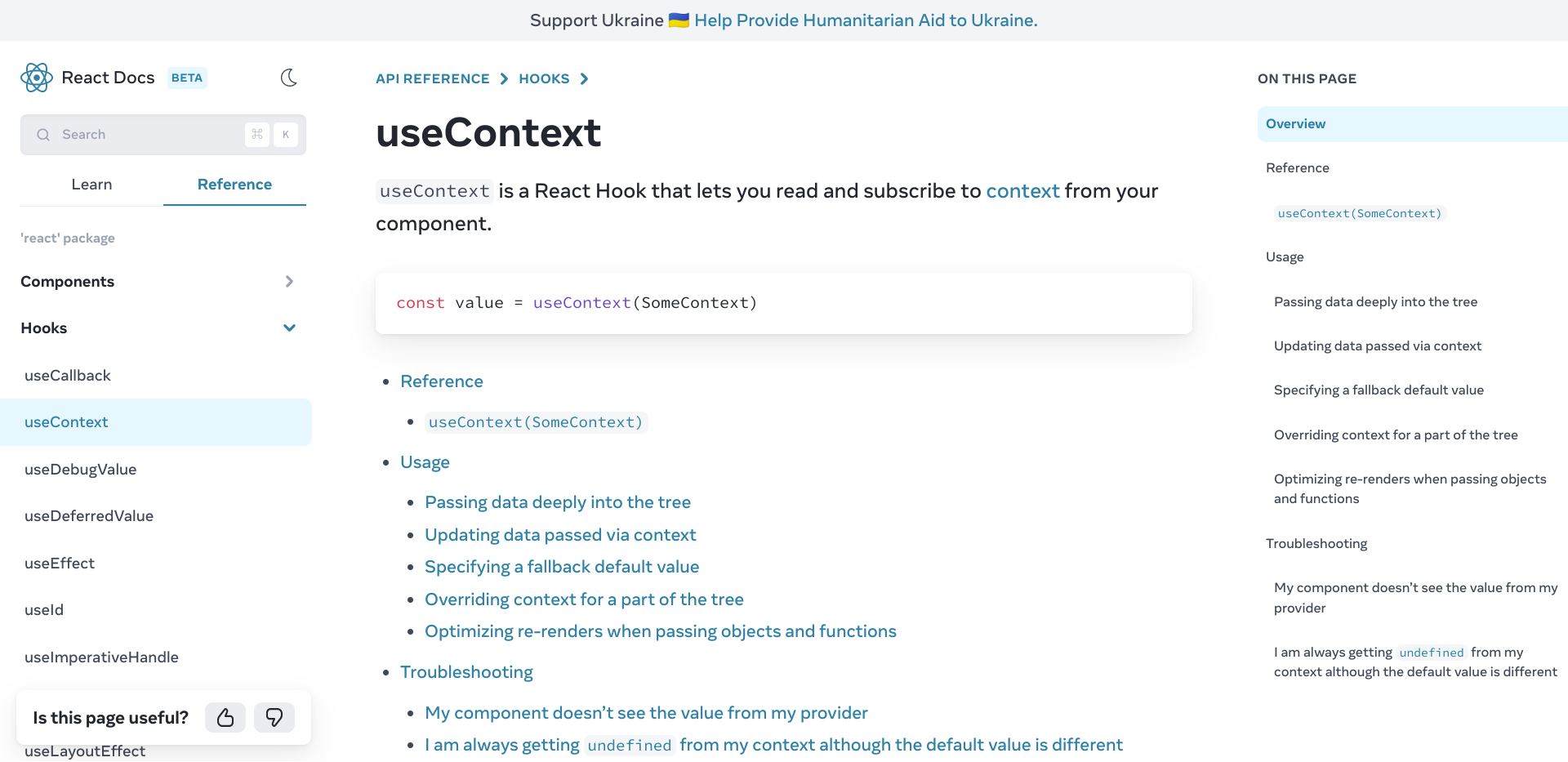
State management is a crucial part of building modern web applications. In React, state refers to the data that drives the behavior of a component. React provides a simple and powerful way to manage state using its built-in state hook. In this blog post, we will explore the basics of state management in React and look at some code examples.
The useState Hook
React provides the useState hook to manage state in functional components. The useState hook returns a pair of values: the current state and a function to update it.
Here's an example of using useState to manage a counter:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
function increment() {
setCount(count + 1);
}
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In this example, we define a functional component called Counter. Inside the component, we call the useState hook and initialize the count state to 0. We also define a function called increment, which updates the count state by calling the setCount function.
In the return statement, we render the current count and a button that calls the increment function when clicked.
Managing Complex State
In many cases, the state of a component can be more complex than a single value. For example, you might have a form with multiple input fields that all need to be managed. In such cases, it's a good idea to use an object or an array to store the state.
Here's an example of using useState to manage an array of items:
import React, { useState } from 'react';
function TodoList() {
const [items, setItems] = useState([]);
function addItem() {
setItems([...items, { text: 'New Item' }]);
}
return (
<div>
<ul>
{items.map((item, index) => (
<li key={index}>{item.text}</li>
))}
</ul>
<button onClick={addItem}>Add Item</button>
</div>
);
}
export default TodoList;
In this example, we define a functional component called TodoList. Inside the component, we call the useState hook and initialize the items state to an empty array. We also define a function called addItem, which updates the items state by appending a new item to the array.
In the return statement, we render the current list of items using the map function and a button that calls the addItem function when clicked.
Using Context
In some cases, you might have state that needs to be shared across multiple components in a React application. In such cases, you can use the React context API to manage the state at a higher level and pass it down to the child components.
Here's an example of using context to manage a user object:
import React, { createContext, useState } from 'react';
const UserContext = createContext();
function App() {
const [user, setUser] = useState({ name: 'John', email: 'john@example.com' });
return (
<UserContext.Provider value={user}>
<Profile />
</UserContext.Provider>
);
}
function Profile() {
const user = useContext(UserContext);
return (
<div>
<p>Name: {user.name}</p>
<p>Email: {user.email}</p>
</div>
);
}
export default App;
In this example, we define a UserContext using the createContext function. Inside the App component, we call the useState hook to initialize the user state to an object with name and email properties. We then wrap the Profile component with the UserContext.Provider and pass the current user object as the value.
Inside the Profile component, we use the useContext hook to access the user object from the context and render the name and email properties.
Using Redux
Redux is a popular state management library that can be used with React. It provides a centralized store for managing state and a set of functions for updating the store.
Here's an example of using Redux to manage a counter:
import React from 'react';
import { createStore } from 'redux';
import { Provider, useSelector, useDispatch } from 'react-redux';
const initialState = { count: 0 };
function reducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
}
const store = createStore(reducer);
function Counter() {
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
function increment() {
dispatch({ type: 'INCREMENT' });
}
function decrement() {
dispatch({ type: 'DECREMENT' });
}
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
function App() {
return (
<Provider store={store}>
<Counter />
</Provider>
);
}
export default App;
In this example, we define a reducer function that takes the current state and an action and returns the new state based on the action. We then create a Redux store using the createStore function and pass the reducer as an argument.
Inside the Counter component, we use the useSelector hook to access the count state from the store and the useDispatch hook to dispatch actions that update the store.
In the App component, we wrap the Counter component with the Provider component and pass the store as a prop.