React Hooks
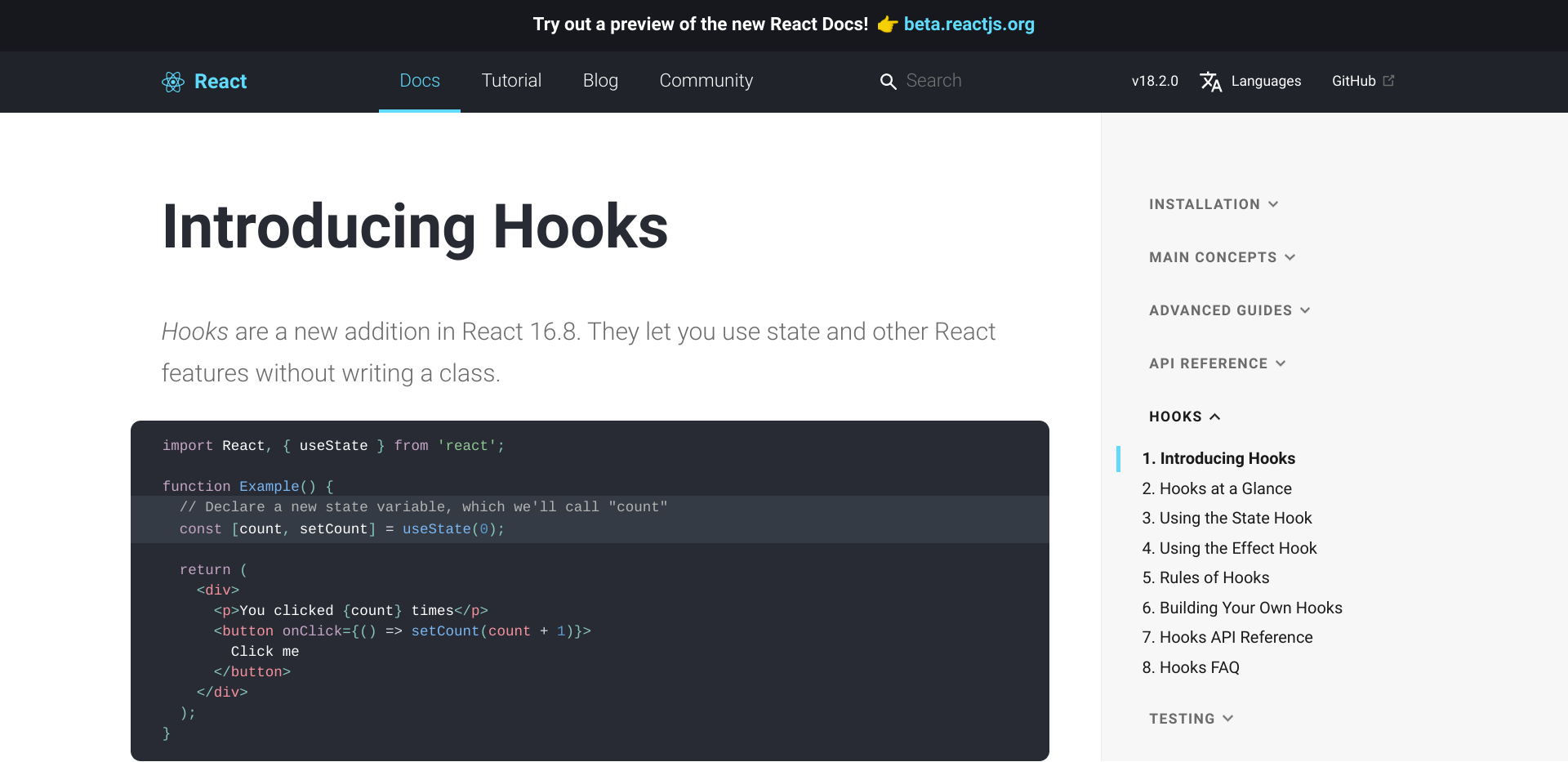
React hooks are a powerful feature introduced in React 16.8. They allow you to use state and other React features without writing a class component. Hooks make it easier to write reusable and testable code, as well as improve the performance of your app.
There are several built-in hooks in React, such as useState, useEffect, and useContext. You can also create custom hooks to reuse logic across components.
Let's look at some code examples to see how to use React hooks.
useState Hook The useState hook allows you to add state to a functional component. It returns an array with two elements: the current state and a function to update the state.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
}
useEffect Hook The useEffect hook allows you to run side effects in a functional component. Side effects include things like fetching data, setting up event listeners, and updating the document title.
import React, { useState, useEffect } from 'react';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => setUsers(data));
}, []);
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
useContext Hook The useContext hook allows you to consume a context in a functional component. Context provides a way to pass data down the component tree without having to pass props manually at every level.
import React, { useContext } from 'react';
import ThemeContext from './ThemeContext';
function Header() {
const theme = useContext(ThemeContext);
return (
<header style={{ background: theme.background, color: theme.text }}>
<h1>My App</h1>
</header>
);
}
Custom Hook You can also create custom hooks to reuse logic across components. Custom hooks are just functions that use other hooks.
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState([]);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(data => setData(data));
}, [url]);
return data;
}
function UserList() {
const users = useFetch('https://jsonplaceholder.typicode.com/users');
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
In this example, the useFetch hook handles fetching data from an API. The UserList component uses the useFetch hook to get a list of users.