State Management in Angular
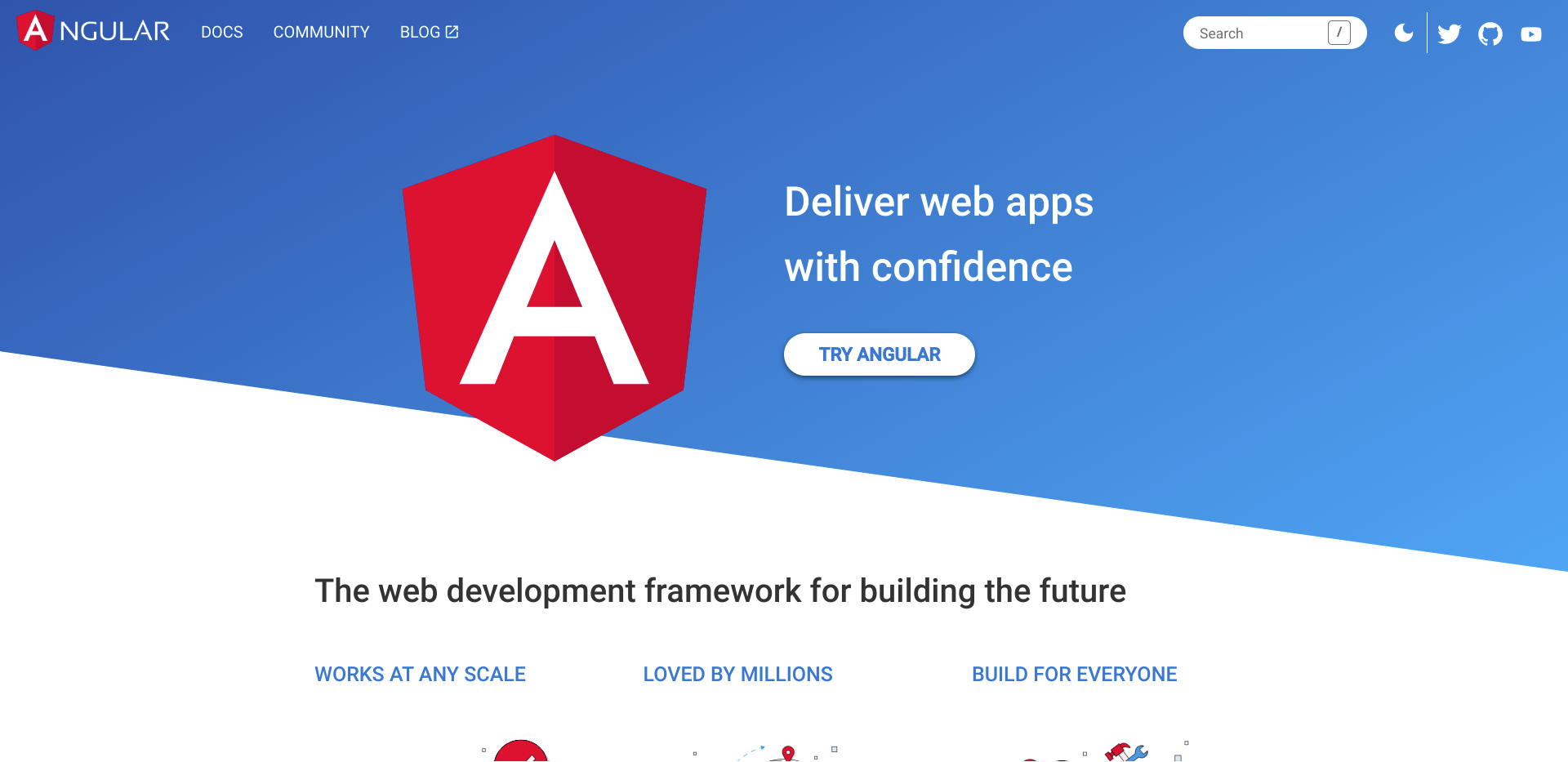
Angular is a powerful and popular web development framework that allows developers to build complex and feature-rich applications with ease. One of the key features of Angular is its powerful state management capabilities, which allow developers to manage complex application states in a clear and scalable way.
State management is the process of managing the data that defines the current state of an application. This can include user input, application configuration settings, and any other data that the application needs to function properly. In Angular, state management is typically accomplished through the use of services, which are classes that encapsulate data and provide methods for manipulating that data.
In this blog post, we will explore state management in Angular and discuss some of the best practices for implementing this functionality in your applications.
In Angular, state management typically involves creating a service that encapsulates application data, and then using that service throughout your application to access and modify that data. For example, consider a simple application that displays a list of todo items:
export class Todo {
constructor(public id: number, public title: string, public completed: boolean) {}
}
@Injectable({ providedIn: 'root' })
export class TodoService {
todos: Todo[] = [
new Todo(1, 'Buy groceries', false),
new Todo(2, 'Walk the dog', false),
new Todo(3, 'Finish Angular tutorial', true),
];
getTodos(): Todo[] {
return this.todos;
}
addTodo(todo: Todo): void {
this.todos.push(todo);
}
removeTodo(todo: Todo): void {
const index = this.todos.indexOf(todo);
if (index !== -1) {
this.todos.splice(index, 1);
}
}
}
In this example, we have defined a Todo
class that represents a single todo item, and a TodoService
class that encapsulates a list of todo items and provides methods for accessing and modifying that list. The getTodos()
method returns the current list of todo items, the addTodo()
method adds a new todo item to the list, and the removeTodo()
method removes a todo item from the list.
To use this service in our application, we can inject it into a component like this:
import { Component } from '@angular/core';
import { TodoService } from './todo.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
constructor(private todoService: TodoService) {}
todos = this.todoService.getTodos();
addTodo(): void {
const newTodo = new Todo(
this.todoService.todos.length + 1,
'New todo item',
false
);
this.todoService.addTodo(newTodo);
this.todos = this.todoService.getTodos();
}
removeTodo(todo: Todo): void {
this.todoService.removeTodo(todo);
this.todos = this.todoService.getTodos();
}
}
In this component, we inject the TodoService
using the constructor, and then use the getTodos()
method to initialize the todos
property. We also define addTodo()
and removeTodo()
methods that use the TodoService
to add and remove todo items from the list, respectively.
When implementing state management in your Angular applications, there are several best practices that you should follow to ensure that your code is clean, maintainable, and scalable.
As we saw in the previous example, it is a good practice to use services to encapsulate application state. This allows you to separate concerns and makes your code more modular and reusable. Services also provide a single source of truth for application state, which makes it easier to manage and maintain.
It is important to use immutable data structures when managing application state in Angular. Immutable data structures cannot be changed once they are created, which makes it easier to track changes and avoid unexpected side effects.
In the previous example, we used the push()
and splice()
methods to modify the todos
array in the TodoService
. Instead of modifying the array directly, we could use methods like concat()
and filter()
to create new arrays that include the desired changes. For example:
addTodo(todo: Todo): void {
this.todos = [...this.todos, todo];
}
removeTodo(todo: Todo): void {
this.todos = this.todos.filter((t) => t.id !== todo.id);
}
In this example, the addTodo()
method creates a new array that includes the existing todos
array plus the new todo
item, and then assigns that new array to the todos
property. Similarly, the removeTodo()
method creates a new array that excludes the todo
item to be removed, and then assigns that new array to the todos
property.
Angular provides an Observable
class that allows you to manage state changes in a reactive way. Observables provide a way to subscribe to changes in data and respond to those changes in real time.
To use observables for state management, you can define a Subject
or BehaviorSubject
in your service and expose it as an observable. For example:
import { BehaviorSubject } from 'rxjs';
@Injectable({ providedIn: 'root' })
export class TodoService {
private todosSubject = new BehaviorSubject<Todo[]>([]);
get todos$(): Observable<Todo[]> {
return this.todosSubject.asObservable();
}
// ...
}
In this example, we define a todosSubject
property that is a BehaviorSubject
that initially contains an empty array. We then define a todos$
property that exposes todosSubject
as an observable. To update the todos
array, we can call the next()
method on todosSubject
:
addTodo(todo: Todo): void {
const updatedTodos = [...this.todosSubject.value, todo];
this.todosSubject.next(updatedTodos);
}
removeTodo(todo: Todo): void {
const updatedTodos = this.todosSubject.value.filter((t) => t.id !== todo.id);
this.todosSubject.next(updatedTodos);
}
In this example, we use the value
property of todosSubject
to get the current array of todos, and then create a new array that includes or excludes the desired changes. We then call the next()
method on todosSubject
to update the observable with the new value.
For complex state management scenarios, it is recommended to use NgRx, a powerful state management library for Angular. NgRx provides a set of reactive state management tools and patterns that allow you to manage complex application states with ease.
NgRx follows the Flux architecture pattern, which separates data flow into a unidirectional flow. It provides a set of building blocks that include actions, reducers, selectors, and effects. These building blocks work together to create a predictable and scalable state management system.