Building a Simple React Component
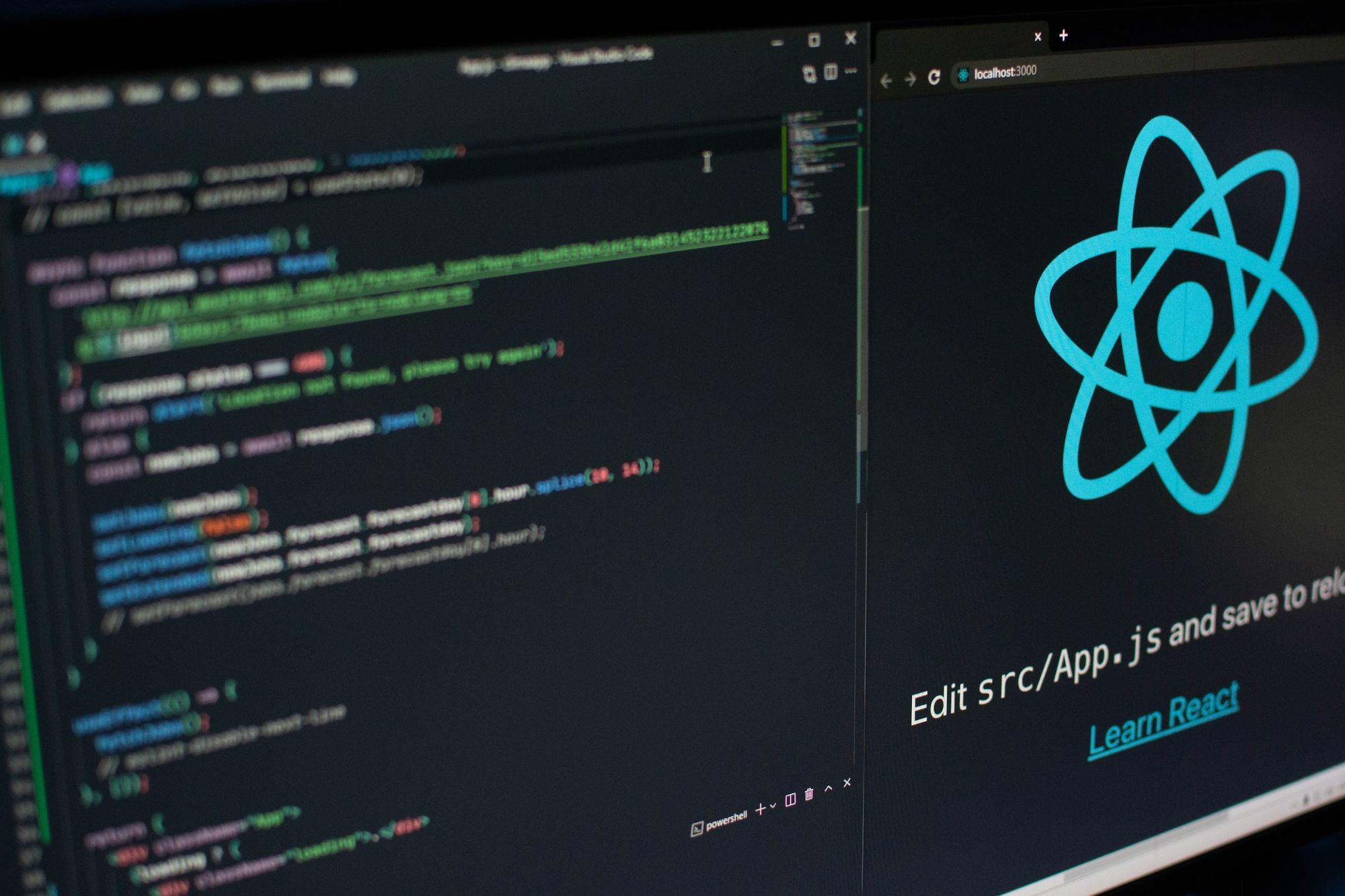
React is a popular JavaScript library for building user interfaces. One of its core features is the ability to create reusable components that can be easily shared and composed to build complex UIs. In this blog post, we will walk through the process of building a simple React component, with code examples to demonstrate each step.
The Component
Our component will be a simple button that displays a message when clicked. Here's what it will look like:
import React from 'react';
function ClickableButton({ message }) {
const handleClick = () => {
alert(message);
};
return (
<button onClick={handleClick}>
Click me
</button>
);
}
export default ClickableButton;
This code defines a new function component called ClickableButton
. It takes a single prop, message
, which is the text that will be displayed when the button is clicked.
The component contains a handleClick
function that uses the alert
function to display the message. It also returns a <button>
element that calls handleClick
when clicked.
Using the Component
Now that we have our component, we can use it in our application. Here's an example of how to do that:
import React from 'react';
import ClickableButton from './ClickableButton';
function App() {
return (
<div>
<h1>My App</h1>
<ClickableButton message="Hello, world!" />
</div>
);
}
export default App;
This code imports our ClickableButton
component and uses it inside a new function component called App
. The App
component returns a <div>
element that contains a <h1>
element and our ClickableButton
component with the message
prop set to "Hello, world!"
.
When we run this code, we should see a button that says "Click me". When we click the button, we should see an alert that says "Hello, world!".